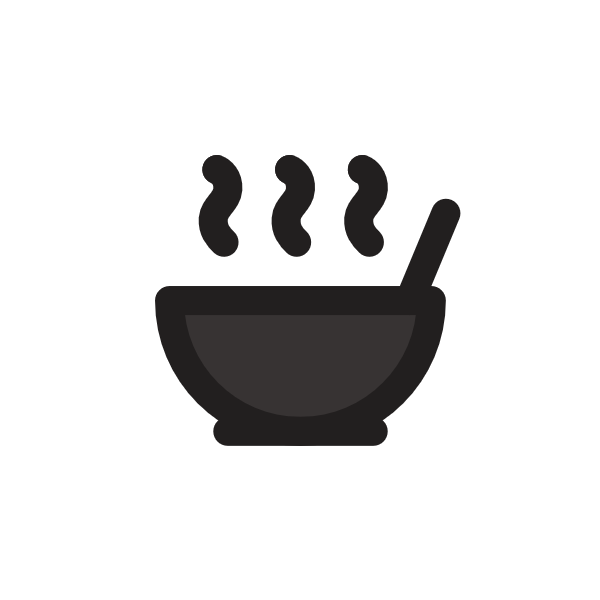
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
C (programming language)
Read the original article here.
C: The Cornerstone for Building Systems from Scratch
Introduction: Why C Matters in Low-Level Computing
In the journey of understanding and building a computer from the ground up, we inevitably move from the raw electrical signals and logic gates of hardware to the software that makes the machine useful. Assembly language provides direct control over the CPU, but writing complex systems entirely in assembly quickly becomes unmanageable. This is where the C programming language becomes indispensable.
Developed in the early 1970s by Dennis Ritchie at Bell Labs, C emerged specifically from the need for a more portable and efficient language to build operating systems – particularly Unix. Its design philosophy was revolutionary: create a language that offers high-level programming conveniences (like structured control flow and data types) while still allowing direct, low-level access to memory and hardware, comparable in power to assembly language, but with significantly improved readability and maintainability.
C is not a language that hides the underlying hardware from you. Instead, it provides constructs that closely mirror the capabilities of the central processing unit (CPU). This "thin abstraction layer" is precisely why C is the language of choice for operating system kernels, device drivers, embedded systems, and performance-critical software – the very components you'd interact with when building a computer "from scratch."
This resource will explore the C language, focusing on the features and historical context that make it essential for anyone diving into the world of low-level systems programming.
Historical Foundation: From Assembly to C
The development of C is intrinsically linked to the creation of the Unix operating system. Early versions of Unix were written largely in assembly language for specific machines like the PDP-7. When the team wanted to port Unix to a new machine, the PDP-11, rewriting the entire operating system in assembly was a daunting task. A higher-level language was needed, but existing languages didn't offer the necessary control over hardware or the desired performance.
Ken Thompson, one of the key figures in Unix development, first created a language called B.
BCPL (Basic Combined Programming Language): An early procedural programming language designed for writing compilers and system software. B was a simplified version of BCPL.
B had limitations; notably, it lacked support for data types other than the machine word (often corresponding to an integer). This made it inefficient for handling characters, which are fundamental for text processing and many system tasks.
Dennis Ritchie enhanced B, adding features like a character data type and richer data types. This evolution, initially called "New B," quickly became the C language we know today. A pivotal moment was the decision to rewrite the Unix kernel itself in C (around 1973 for Version 4 Unix). This demonstrated C's capabilities as a systems programming language and significantly improved the portability of Unix – a C compiler could be written for a new machine, and then the bulk of the OS could be compiled on that new hardware.
Unix Kernel: The core component of the Unix operating system, responsible for managing system resources like memory, processes, and hardware. Rewriting it in C was a landmark achievement, proving that complex operating systems could be built in a high-level language.
The need for consistency across different implementations led to formal standardization processes.
- K&R C (circa 1978): Defined by the first edition of "The C Programming Language" by Brian Kernighan and Dennis Ritchie. This book served as the de facto standard for many years. K&R C was more permissive than later standards, for instance, implicitly assuming
int
as a return type if none was specified. - ANSI C / ISO C (C89/C90, 1989/1990): The first formal standard, established by ANSI and later adopted by ISO. It codified many common practices and added crucial features like function prototypes (improving type checking) and the
void
pointer. This brought much-needed rigor and portability. - Later Standards (C99, C11, C17, C23): Subsequent revisions have added features like
long long int
, complex numbers, variable-length arrays, improved floating-point support, multi-threading capabilities, and more. While modern development often uses these, C89 remains a fundamental baseline for portability.
Understanding this history reveals C's fundamental design goal: to be a powerful tool for building the very foundation of computing systems.
Core Language Concepts
C is characterized by its imperative, procedural nature, strong tie to hardware, and minimal runtime requirements.
Imperative Programming: A programming paradigm that uses statements to change a program's state. C code consists of a sequence of commands for the computer to execute.
Procedural Programming: A type of imperative programming based on the concept of the procedure call. Programs are structured into procedures (or functions) that perform specific tasks.
Static Type System: Types of variables are checked at compile time rather than at runtime. This helps catch certain errors early in the development process.
Runtime Support: The minimal code that needs to run alongside your compiled program to make it work (e.g., memory management, garbage collection, virtual machine). C has very little runtime support; much is handled by the compiled code itself or standard library functions.
Program Structure and Syntax
A C program is typically composed of one or more source files (.c
files) and header files (.h
files). Source files contain function definitions and variable declarations, while header files primarily contain declarations and macros used across multiple source files.
- Free-Form: C code isn't bound to specific line numbers or indentation (though good style is crucial for readability).
- Statements: Actions are expressed as statements, terminated by a semicolon (
;
). - Blocks: Multiple statements can be grouped into a single block using curly braces (
{}
). Blocks define scope for variables. - Keywords: A small, fixed set of reserved words have predefined meanings (e.g.,
int
,if
,while
,return
).
Control Flow
C provides standard constructs for controlling the order of execution:
- Conditional Execution:
if
,else
,switch
if (temperature > 100) { // Code to run if condition is true } else { // Code to run otherwise } switch (command) { case 'A': // Execute command A break; // Prevent fall-through case 'B': // Execute command B // Falls through to default if no break default: // Execute default case }
- Iterative Execution (Loops):
for
,while
,do...while
// For loop: Initialization; Condition; Update for (int i = 0; i < 10; i++) { // Code to repeat 10 times } // While loop: Condition checked before each iteration while (data_available) { // Process data } // Do-while loop: Code executes at least once, condition checked after do { // Get input } while (input_invalid);
- Jumps:
break
,continue
,goto
(usegoto
sparingly, as it can make code hard to follow)// Inside a loop: if (error) break; // Exit the loop if (skip_item) continue; // Skip to the next iteration
Operators
C is known for its rich set of operators, including arithmetic, bitwise, logical, comparison, assignment, and pointer operators. These map closely to typical CPU instructions.
- Arithmetic:
+
,-
,*
,/
,%
(modulo) - Assignment:
=
,+=
,-=
,*=
,/=
,%=
,&=
,|=
,^=
,<<=
,>>=
- Bitwise:
~
(NOT),&
(AND),|
(OR),^
(XOR),<<
(left shift),>>
(right shift)- Context for "Building from Scratch": Bitwise operators are fundamental for manipulating individual bits or groups of bits, essential when dealing with hardware registers, flags, or packed data structures. Understanding how these map to CPU instructions is key.
- Logical:
!
(NOT),&&
(AND),||
(OR) - Comparison:
==
,!=
,<
,<=
,>
,>=
- Pointer/Address:
&
(address-of),*
(dereference),[ ]
(array subscripting, often seen as pointer arithmetic) - Other:
sizeof
(determines size of a type or object),(type)
(type cast),,
(sequence point)
Operator Precedence and Associativity: C has strict rules about the order in which operators are evaluated (
*
before+
,&&
after==
, etc.). This is crucial but can be unintuitive, sometimes requiring parentheses to ensure the desired evaluation order. Example:x & 1 == 0
is parsed asx & (1 == 0)
, not(x & 1) == 0
.
Functions
Code in C is organized into functions. Every executable C program must have a main
function, which is the entry point.
- Definition:
return_type function_name(parameter_list) { /* code */ }
- Return Value: Functions can return a single value.
void
is used for functions that don't return a value (procedures). - Parameters: Parameters are passed by value. A copy of the argument's value is passed to the function. To simulate pass-by-reference, you pass a pointer to the data.
- No Nested Functions: Functions cannot be defined inside other functions.
- Recursion: Functions can call themselves.
Pass-by-Value: When you pass a variable to a function, the function receives a copy of that variable's value. Changes made to the parameter inside the function do not affect the original variable outside the function.
Pass-by-Reference (Simulated): By passing a pointer to a variable, the function receives the memory address of the original variable. Using the dereference operator (
*
), the function can access and modify the data at that memory address, thus affecting the original variable.
Data Types: Shaping the Bits
C's type system provides a way to interpret sequences of bits in memory. It's static but weakly enforced, allowing for explicit control over how data is treated.
- Built-in Types:
- Integers:
char
,short
,int
,long
,long long
. These come insigned
andunsigned
variants. The exact size of these types can vary depending on the system's architecture (e.g.,int
is often 32-bit on modern systems, but could be 16-bit on older ones).- Context for "Building from Scratch": The size of these types is machine-dependent. Understanding the architecture's word size, bus width, and how C types map to these is crucial for low-level development.
char
is typically 1 byte, often used for representing bytes when dealing with raw memory or hardware registers.
- Context for "Building from Scratch": The size of these types is machine-dependent. Understanding the architecture's word size, bus width, and how C types map to these is crucial for low-level development.
- Floating-Point:
float
,double
,long double
. For representing numbers with decimal points. C99 added acomplex
type. - Boolean: C99 introduced
_Bool
(often used via the<stdbool.h>
header with thebool
synonym). Before this, integers (0
for false, non-zero for true) were used. - Enumerated Types (
enum
): Allow creating a set of named integer constants.
- Integers:
- Derived Types:
- Arrays: Collections of elements of the same type, stored contiguously in memory.
- Pointers: Variables that store memory addresses.
- Structs (
struct
): Allow grouping variables of different types under a single name. Useful for representing records or complex data structures.- Context for "Building from Scratch": Structs are vital for defining the layout of data in memory, crucial for interacting with hardware registers (which often have a defined bit layout), file formats, or network packets.
- Unions (
union
): Allow multiple variables to share the same memory location. Only one member can be active at a time.- Context for "Building from Scratch": Unions are often used for type punning (interpreting the same bits in memory as different types) or saving memory when only one of several possible data types is needed at a time.
Type Casting: Explicitly converting a value from one type to another (e.g.,
(float) my_int
). This tells the compiler how to reinterpret the bits.Usual Arithmetic Conversions: C has rules for automatically converting types in expressions (e.g., an
int
might be promoted to afloat
before an operation). This can sometimes lead to unexpected results, especially when mixing signed and unsigned types.
Pointers: The Power of Address Manipulation
Pointers are one of C's most powerful and potentially dangerous features. They are fundamental to C's ability to interact directly with memory, which is essential in systems programming.
Pointer: A variable that stores the memory address of another variable or a function.
Dereferencing (
*
): Accessing the value stored at the memory address held by a pointer. The expression*ptr
gives you the value at the addressptr
points to.Address-of (
&
): An operator used to get the memory address of a variable. The expression&var
gives you the address wherevar
is stored.
How Pointers are Used:
- Accessing Variables Indirectly: Functions can modify variables outside their local scope by taking a pointer as an argument (simulating pass-by-reference).
- Dynamic Memory Allocation: Functions like
malloc
,calloc
, andrealloc
(from the standard library) return pointers to blocks of memory allocated from the heap at runtime. Pointers are used to access and manage this memory. - Data Structures: Linked lists, trees, graphs, and other complex data structures are built by linking nodes together using pointers.
- Arrays: Array names often decay into pointers to their first element. Pointer arithmetic is the underlying mechanism for array indexing (
array[i]
is equivalent to*(array + i)
). - Interacting with Hardware: Memory-mapped I/O involves accessing hardware registers at specific memory addresses. C pointers are used to read from or write to these addresses directly.
Pointer Arithmetic: C allows adding or subtracting integers to pointers. When you add N
to a pointer of type T*
, the compiler automatically scales N
by sizeof(T)
. This means ptr + 1
points to the next element of type T
in memory, not just the next byte. This scaling is a key feature that makes pointer arithmetic safe and convenient for traversing arrays and data structures.
Special Pointer Types:
- Null Pointer: A pointer explicitly set to point to no valid memory location. Represented by the integer constant
0
or theNULL
macro. Dereferencing a null pointer is a common source of errors (often causing a "segmentation fault" on systems with memory protection). - Void Pointer (
void *
): A generic pointer that can point to data of any type. Useful for functions that need to work with arbitrary data (like memory allocation functions or data structure libraries). Avoid *
cannot be directly dereferenced or used in pointer arithmetic; it must be cast to a pointer of a specific type first.
Dangers of Pointers:
While powerful, pointers are a primary source of bugs in C:
- Dangling Pointers: A pointer that points to memory that has been deallocated.
- Wild Pointers: An uninitialized pointer that points to an arbitrary, potentially unsafe memory location.
- Illegal Access: Using pointer arithmetic or casting to access memory outside the intended object or allocated block.
- Buffer Overflows: Writing past the end of an allocated buffer via pointer manipulation or array indexing.
These issues highlight that C places the burden of memory safety and pointer correctness squarely on the programmer. This level of control is necessary for systems programming but requires careful discipline.
Arrays: Ordered Collections
Arrays in C are fixed-size, contiguous blocks of memory holding elements of the same data type. C99 introduced variable-length arrays (VLAs), whose size can be determined at runtime, but traditional arrays have their size fixed at compile time.
int my_array[10]; // Declares an array of 10 integers
my_array[0] = 1; // Accessing the first element (index 0)
my_array[9] = 2; // Accessing the last element (index 9)
// my_array[10] = 3; // ERROR: Out of bounds access!
Array-Pointer Interchangeability: In most contexts, the name of an array (
my_array
) automatically "decays" into a pointer to its first element (&my_array[0]
). This is why you can often pass arrays to functions using pointer syntax, and why array subscripting (arr[i]
) is equivalent to pointer arithmetic (*(arr + i)
).
While arrays are contiguous, C does not typically perform automatic bounds checking when you access array[i]
. Accessing memory outside the declared bounds of the array (e.g., my_array[10]
in the example above) leads to undefined behavior, which can result in crashes, data corruption, or security vulnerabilities (like buffer overflows). This is another aspect where C prioritizes performance and low-level control over built-in safety.
Multi-dimensional arrays are implemented as arrays of arrays (e.g., int matrix[3][4];
). matrix[i][j]
is syntactic sugar that the compiler translates using pointer arithmetic based on the dimensions.
Memory Management: Your Responsibility
C offers programmers explicit control over memory allocation and deallocation, a crucial capability for systems programming where resources are finite and predictability is paramount.
Static Memory Allocation: Memory allocated at compile time. Global variables and
static
variables within functions reside in static memory. This memory exists for the entire duration of the program's execution.Automatic Memory Allocation: Memory allocated on the call stack when a function is entered or a block is entered. Local variables (unless
static
) use automatic allocation. This memory is automatically freed when the function returns or the block is exited.Dynamic Memory Allocation: Memory allocated from the heap at runtime using functions like
malloc
,calloc
,realloc
, and freed usingfree
(all from the standard library, typically in<stdlib.h>
). This allows allocating memory whose size is not known until the program is running and managing its lifetime explicitly.
- Static: Predictable, no overhead at runtime, fixed size, exists for program lifetime. Used for global configuration data, constant strings.
- Automatic: Simple to use, automatically managed, limited size (stack overflow is possible), transient. Used for most local variables and function parameters.
- Dynamic: Flexible size, persists until explicitly freed, potential for significant runtime overhead, requires careful management. Used for data structures that grow or shrink, data whose size depends on input (like reading a file into memory).
Heap: A region of memory available for dynamic allocation. Unlike the stack, which is managed automatically, the heap requires explicit calls to allocation and deallocation functions.
Stack: A region of memory used for automatic allocation. Function calls and local variables are pushed onto the stack; they are popped off when the function returns. The stack grows and shrinks automatically.
The explicit nature of dynamic memory management in C is powerful but is also a major source of bugs:
- Memory Leaks: Failure to
free
dynamically allocated memory when it's no longer needed. The memory remains allocated, reducing available memory over time and potentially crashing long-running programs or systems. - Dangling Pointers: Using a pointer after the memory it pointed to has been freed. Accessing freed memory leads to undefined behavior.
- Double Free: Attempting to free the same block of memory more than once. Also leads to undefined behavior.
Languages with automatic garbage collection handle dynamic memory cleanup automatically, but C's manual approach gives the programmer precise control, which is critical in environments with limited memory or strict timing requirements, like embedded systems or operating system kernels where a garbage collector might be unacceptable.
The C Preprocessor: Manipulating Source Code
Before the C compiler translates your source code into machine code, the C preprocessor performs text transformations on the source file. Directives begin with a #
symbol.
Preprocessor: A program that processes the source code before it is passed to the compiler. It handles directives like
#include
,#define
, and conditional compilation.
Key preprocessor features:
- File Inclusion (
#include
): Inserts the content of another file (usually a header file) into the current source file. Angle brackets (< >
) typically search system header directories, while double quotes (" "
) search local directories first.- Context for "Building from Scratch": Header files are used to declare functions and variables defined in other source files or libraries (
.h
files define what is available,.c
files provide the actual implementation). They are essential for structuring larger programs and using libraries.
- Context for "Building from Scratch": Header files are used to declare functions and variables defined in other source files or libraries (
- Macro Definition (
#define
): Defines symbolic constants or simple text substitutions. Macros can be plain substitutions or take arguments (like functions, but processed as text).#define BUFFER_SIZE 1024 // Text substitution #define MAX(a, b) ((a) > (b) ? (a) : (b)) // Macro with arguments
- Context for "Building from Scratch": Macros are heavily used for defining hardware-specific addresses or values (e.g.,
#define UART_BASE_ADDR 0x1000
), bit masks, or configuration options that need to be set before compilation.
- Context for "Building from Scratch": Macros are heavily used for defining hardware-specific addresses or values (e.g.,
- Conditional Compilation (
#ifdef
,#ifndef
,#if
,#else
,#elif
,#endif
): Allows including or excluding blocks of code based on whether certain macros are defined or specific conditions are true.#ifdef DEBUG printf("Debug message\n"); // This line is only compiled if DEBUG is defined #endif #if PROCESSOR_ARCH == ARM // ARM-specific code #elif PROCESSOR_ARCH == X86 // X86-specific code #endif
- Context for "Building from Scratch": Essential for writing portable code that needs to adapt to different hardware architectures, operating systems, or build configurations. You can include specific drivers or code paths based on compilation flags.
The preprocessor works purely on text, unaware of C syntax. This can lead to subtle errors if not used carefully, but its power in allowing source code transformation is invaluable in low-level contexts.
Modularity and Libraries
C supports modular programming by allowing code to be split across multiple source files.
Compilation Unit: A single source file (
.c
) after preprocessing. Each compilation unit is typically compiled independently into an object file (.o
or.obj
).Object File: Contains machine code for the functions and data defined in a single compilation unit, along with symbols (names of functions and variables) that can be referenced by other code.
Linker: A tool that takes one or more object files and libraries and combines them into a single executable program or library. It resolves references between object files, ensuring that function calls and variable accesses point to the correct locations.
static
keyword: Used within a file to restrict the visibility of functions or global variables to only that file. This helps prevent naming conflicts between different modules.extern
keyword: Used in a header file or source file to declare that a function or variable is defined in another compilation unit. This tells the compiler that the linker will resolve the reference later.
Library: A collection of pre-compiled object code (often from multiple source files) that can be linked with programs. Libraries provide reusable functionality.
The most critical library in C is the C Standard Library.
C Standard Library: A collection of standard header files and pre-compiled functions specified by the C standard. It provides essential, portable functionalities such as input/output (stdio.h), memory allocation (stdlib.h), string manipulation (string.h), mathematical functions (math.h), etc.
- Input/Output (I/O): Handled via the standard library, particularly
<stdio.h>
, using the concept of streams. This abstracts away the underlying hardware details (like disks, keyboards, screens) and presents them as sequences of bytes.Stream: An abstract source or destination of data (e.g., a file, a keyboard input, a screen output). The standard library buffers stream I/O for efficiency.
- System Interfaces: While not part of the core C standard library, operating systems (like Unix/Linux, Windows) provide extensive libraries accessible from C that allow programs to interact with the OS kernel for tasks like file system access, process management, networking, and low-level device control. POSIX is a standard specifying many of these interfaces for Unix-like systems.
The library model means the core C language is relatively small. Complex tasks are delegated to libraries, which can be swapped or customized depending on the target environment (e.g., a standard OS vs. a bare-metal embedded system).
Why C is Essential for Systems Programming
Recapping and expanding on the initial point, C's dominance in systems programming stems from its unique combination of features:
- Low-Level Memory Access: Pointers and the ability to cast between pointer types allow direct manipulation of memory addresses. This is indispensable for interacting with hardware registers (memory-mapped I/O), implementing memory managers, and manipulating data structures without the overhead of higher-level abstractions.
- Minimal Runtime: C programs require very little support code to run. Once compiled, the executable is largely just the machine code translated from your C source, plus code from linked libraries. There's no large virtual machine or complex runtime environment needed. This makes C suitable for environments with extremely limited resources (like tiny microcontrollers) or where the runtime itself needs to be built (like an operating system kernel).
- Predictable Performance: C's close relationship to hardware means compiled code often maps efficiently to machine instructions. The manual memory management provides predictable allocation and deallocation times, avoiding the potentially unpredictable pauses introduced by automatic garbage collection.
- Hardware Feature Exposure: C's operators (especially bitwise) and data types allow programmers to directly leverage CPU capabilities. For specialized instructions or hardware, C compilers often provide intrinsic functions or support assembly language embedding.
- Modularity and Linking: The separate compilation and linking model facilitates building complex systems from smaller, manageable modules. C's calling conventions and linking format are often the standard that other languages adhere to, making C code callable from and callable by code written in assembly or other languages.
- Widespread Availability and Ecosystem: C compilers (
gcc
,clang
,MSVC
, etc.) exist for virtually every CPU architecture imaginable, from the smallest 8-bit microcontrollers to the largest supercomputers. This vast ecosystem of compilers, debuggers, tools, and libraries makes C a practical choice even today.
Challenges and Considerations
Despite its power and suitability for low-level tasks, C is not without its difficulties, many of which are direct consequences of the control it grants the programmer:
- Manual Memory Management: As discussed, managing memory dynamically with
malloc
andfree
is prone to errors like memory leaks, dangling pointers, and double frees. These are hard bugs to track down. - Lack of Built-in Safety Checks: C generally doesn't perform runtime checks for things like array bounds, null pointer dereferences, or type correctness in many situations. This means programmer errors can lead to crashes, security vulnerabilities (like buffer overflows), and data corruption without explicit warnings or errors from the compiler or runtime.
- Weak Typing: While static, C's type system is relatively weak, allowing implicit conversions and easy circumvention via casting or unions. This can obscure programmer intent and lead to unexpected behavior.
- Undefined Behavior: C's standard leaves certain actions (like dereferencing a null pointer, accessing memory out of bounds, or relying on evaluation order within expressions) as "undefined behavior." This means the compiler is free to do anything, from crashing the program to appearing to work correctly, potentially varying between compilers or optimization levels. Writing portable and robust C code requires careful avoidance of undefined behavior.
- Low-Level Abstractions: C lacks built-in support for higher-level concepts common in modern languages, such as object-oriented programming, exceptions, generics, or automatic garbage collection. These must be implemented manually or through libraries, adding complexity.
- String Handling: Strings are simply null-terminated arrays of characters. Most string operations require manual memory management and careful handling of array bounds, contrasting with languages that have dedicated, safer string types.
For systems programming, these aren't necessarily seen as fatal flaws but as trade-offs for performance and control. They highlight the discipline required to write correct and robust C code. Specialized coding standards like MISRA C or CERT C exist to mitigate some of these risks in safety-critical applications.
Relationship with Other Languages
C has profoundly influenced the landscape of programming languages. Many languages borrow its syntax, control structures, and operator set.
- C++: Originally designed as "C with Classes," C++ is a superset of C that adds object-oriented programming features, stronger typing, templates for generic programming, and more sophisticated standard libraries. While mostly compatible, there are subtle differences between C and C++.
- Objective-C: Another object-oriented extension of C, popular in Apple's ecosystem. It combines C's syntax with Smalltalk-style messaging.
- Other Influences: Languages like Java, C#, JavaScript, Perl, Python, PHP, Ruby, Swift, and Go all show syntactic or conceptual influence from C.
Furthermore, C's efficiency and ubiquity mean that compilers, interpreters, and runtime environments for many other languages (like Python, Perl, Ruby, PHP) are themselves often implemented in C. C also serves as a common target or intermediate language for compilers of other languages.
Conclusion: C's Enduring Relevance
For the aspiring builder of computers and operating systems from scratch, understanding C is not just recommended; it is almost a prerequisite. C provides the essential bridge between the raw capabilities of hardware and the complex layers of software that run on it.
Its design, born from the practical needs of building an operating system, offers the necessary low-level control over memory and hardware, coupled with structured programming features that allow for the creation of complex systems without falling back entirely to assembly language. While it demands careful attention to detail and manual management of resources, this very control is what makes it powerful for tasks where performance, predictability, and direct hardware interaction are paramount.
By learning C, you gain insight into how operating systems manage memory, how device drivers communicate with hardware, and how high-level applications interact with the system foundation. It is a challenging language, but mastering C opens the door to understanding the core software layers that make hardware functional – a vital skill in the lost art of building a computer from scratch.
Related Articles
See Also
- "Amazon codewhisperer chat history missing"
- "Amazon codewhisperer keeps freezing mid-response"
- "Amazon codewhisperer keeps logging me out"
- "Amazon codewhisperer not generating code properly"
- "Amazon codewhisperer not loading past responses"
- "Amazon codewhisperer not responding"
- "Amazon codewhisperer not writing full answers"
- "Amazon codewhisperer outputs blank response"
- "Amazon codewhisperer vs amazon codewhisperer comparison"
- "Are ai apps safe"